Receiving messages
State | iOS | Android |
Foreground | ✅ | ✅ |
Background | ✅ | ✅ |
Terminated | ✅ | ✅ |
Recipients
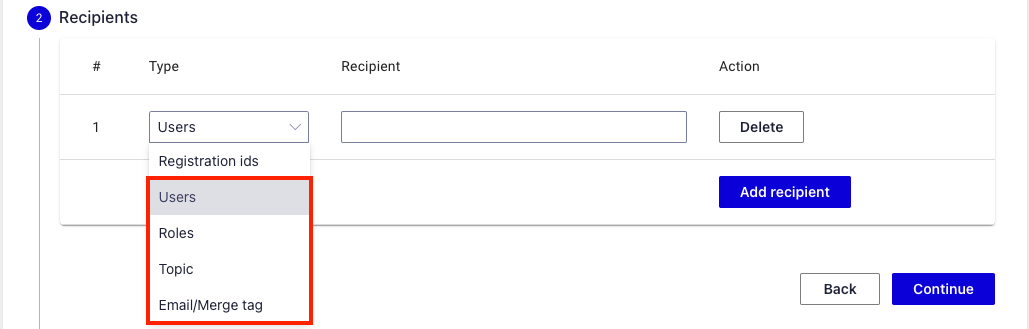
- Registration ids
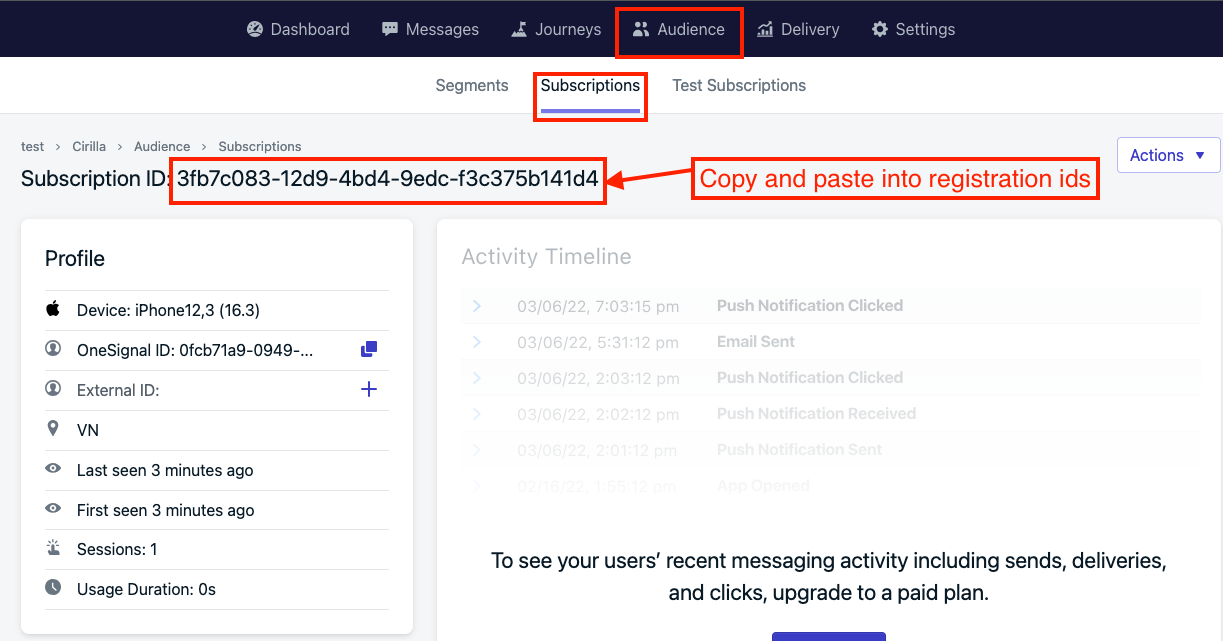
Platform Setup
Admin Setup
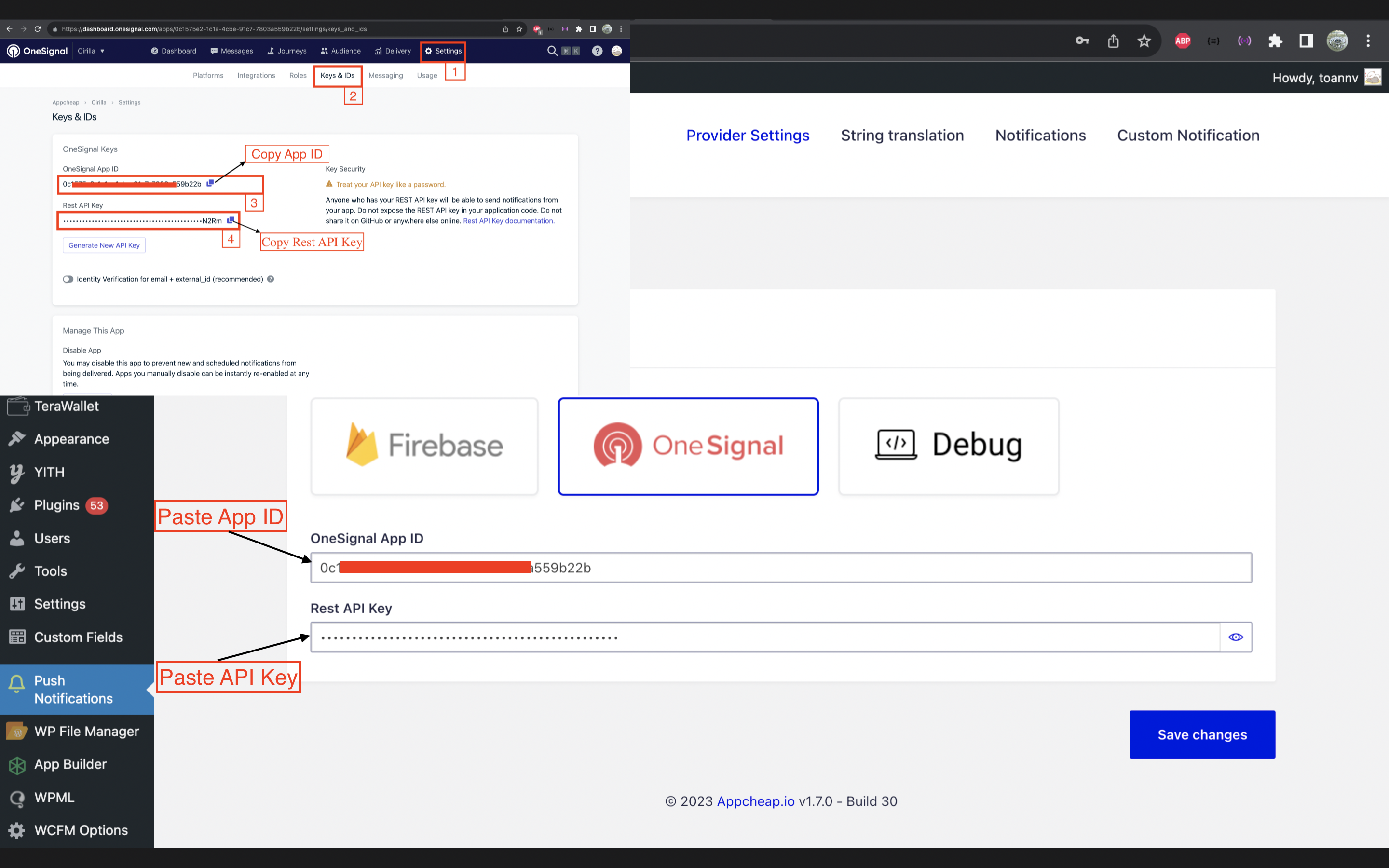
Install Package
1: Open: cirilla/pubspec.yaml
, and paste the following inside of it:
onesignal_flutter: ^3.5.1
Result:
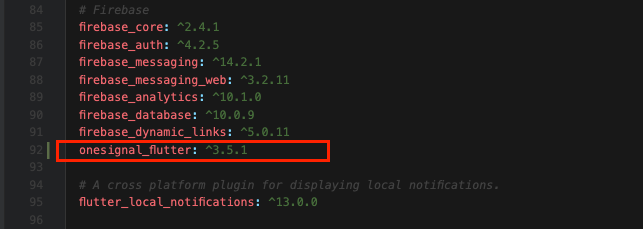
2: Open terminal, cd
to the cirilla project, and run flutter pub get
iOS Setup
1: Open: cirilla/ios/Podfile
, and paste the following inside of it:
pod 'OneSignalXCFramework', '>= 3.0.0', '< 4.0'
Result:
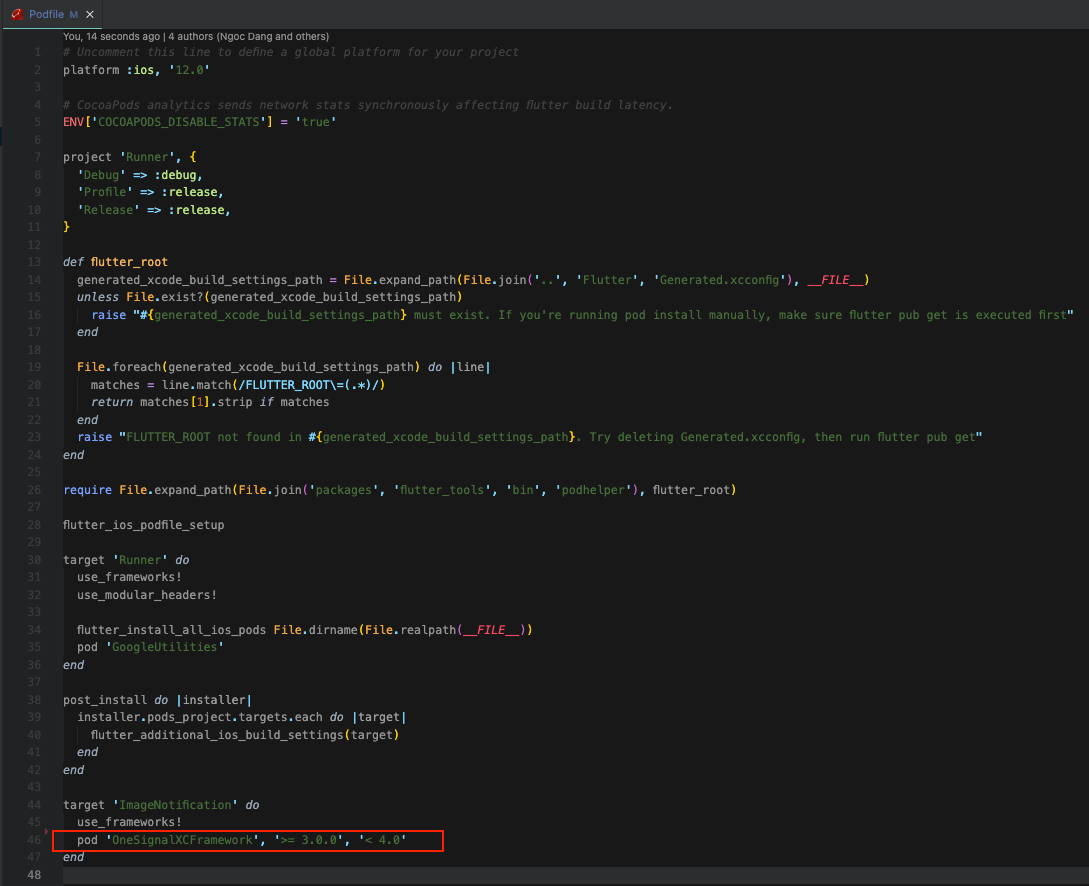
2: Open terminal, cd
to the ios
directory, and run pod install
3: Open: cirilla/ios/ImageNotification/NotificationService.m
, and paste the following inside of it:
//
// NotificationService.m
// ImageNotification
//
// Created by Dang Ngoc on 05/08/2022.
//
#import "NotificationService.h"
#import <OneSignal/OneSignal.h>
@interface NotificationService ()
@property (nonatomic, strong) void (^contentHandler)(UNNotificationContent *contentToDeliver);
@property (nonatomic, strong) UNNotificationRequest *receivedRequest;
@property (nonatomic, strong) UNMutableNotificationContent *bestAttemptContent;
@end
@implementation NotificationService
- (void)didReceiveNotificationRequest:(UNNotificationRequest *)request withContentHandler:(void (^)(UNNotificationContent * _Nonnull))contentHandler {
self.receivedRequest = request;
self.contentHandler = contentHandler;
self.bestAttemptContent = [request.content mutableCopy];
[OneSignal didReceiveNotificationExtensionRequest:self.receivedRequest
withMutableNotificationContent:self.bestAttemptContent
withContentHandler:self.contentHandler];
}
- (void)serviceExtensionTimeWillExpire {
// Called just before the extension will be terminated by the system.
// Use this as an opportunity to deliver your "best attempt" at modified content, otherwise the original push payload will be used.
[OneSignal serviceExtensionTimeWillExpireRequest:self.receivedRequest withMutableNotificationContent:self.bestAttemptContent];
self.contentHandler(self.bestAttemptContent);
}
@end
Result:
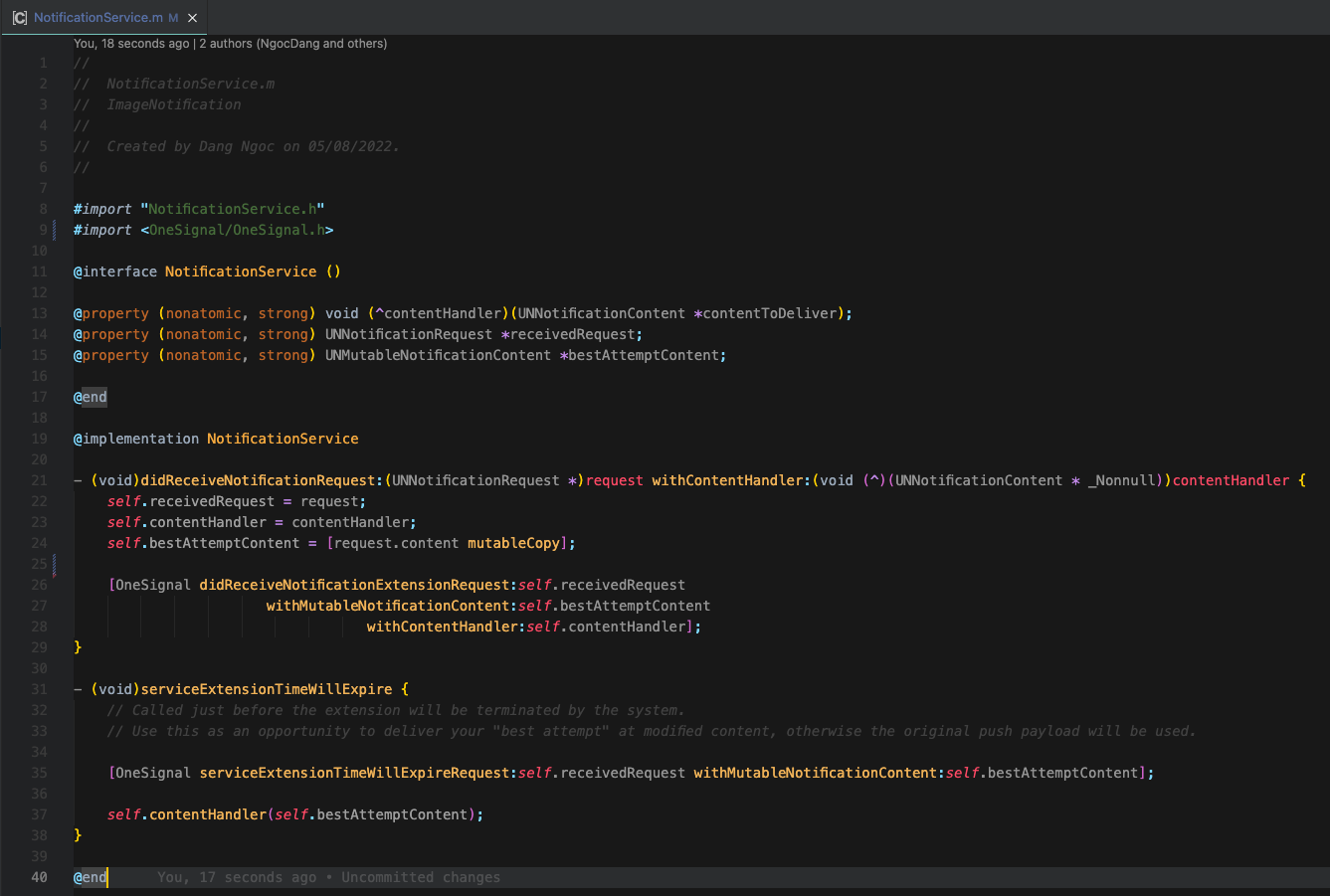
Messaging File Setup
Note: copy the app id and paste it to replace the code “xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx
“.
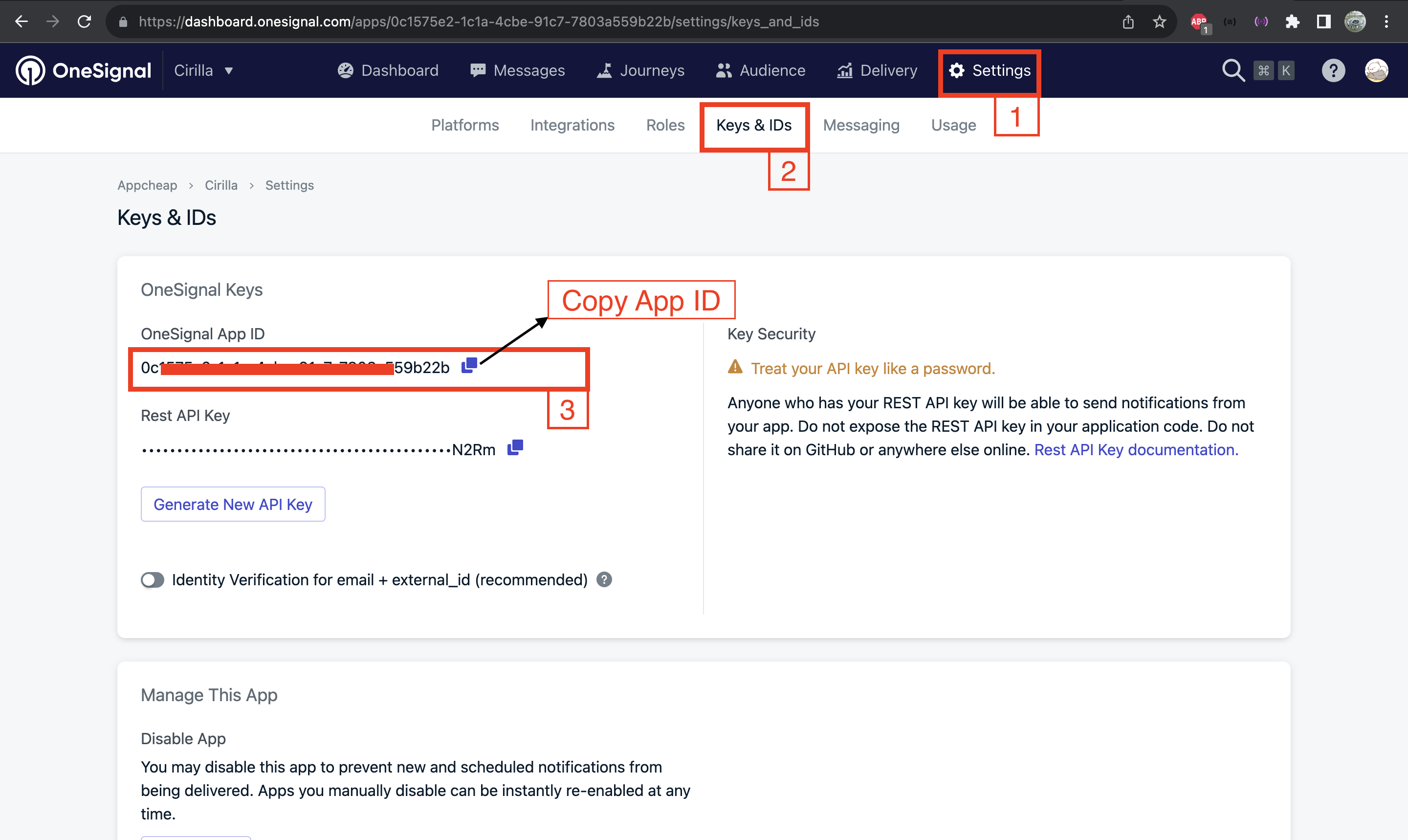
Result:
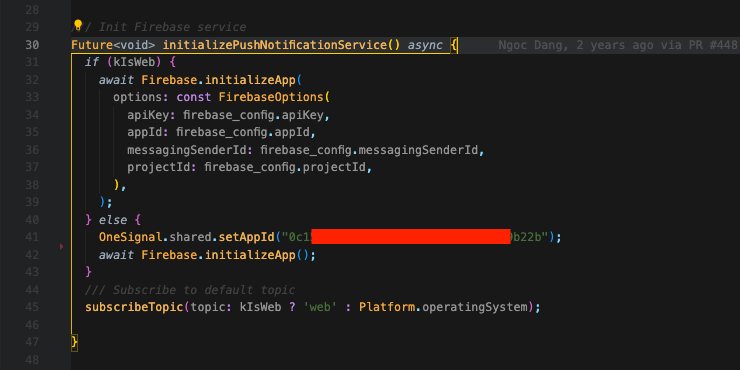
Open: cirilla/lib/service/messaging.dart
, and paste the following inside of it:
import 'dart:async';
import 'dart:convert';
import 'dart:io';
import 'package:cirilla/constants/constants.dart';
import 'package:cirilla/service/service.dart';
import 'package:cirilla/utils/debug.dart';
import 'package:firebase_core/firebase_core.dart';
import 'package:flutter/material.dart';
import 'package:flutter/foundation.dart';
import 'package:path_provider/path_provider.dart';
import 'package:shared_preferences/shared_preferences.dart';
import '../constants/firebase_options.dart' as firebase_config;
import 'package:onesignal_flutter/onesignal_flutter.dart';
SharedPreferences? sharedPref;
Future<SharedPreferences> getSharedPref() async {
sharedPref ??= await SharedPreferences.getInstance();
if (!isWeb) {
final Directory appDocDir = await getApplicationDocumentsDirectory();
await sharedPref?.setString('appDocDir', appDocDir.path);
}
return sharedPref!;
}
/// Init Firebase service
Future<void> initializePushNotificationService() async {
if (kIsWeb) {
await Firebase.initializeApp(
options: const FirebaseOptions(
apiKey: firebase_config.apiKey,
appId: firebase_config.appId,
messagingSenderId: firebase_config.messagingSenderId,
projectId: firebase_config.projectId,
),
);
} else {
OneSignal.shared.setAppId("xxxxxxxx-xxxx-xxxx-xxxx-xxxxxxxxxxxx");
await Firebase.initializeApp();
}
/// Subscribe to default topic
subscribeTopic(topic: kIsWeb ? 'web' : Platform.operatingSystem);
}
/// Update token to database
Future<void> updateTokenToDatabase(
RequestHelper requestHelper,
String? token, {
List<String>? topics,
String? userId,
String? email,
}) async {
try {
if (topics != null) {
await subscribeTopic(topic: topics, userId: userId, email: email);
}
} catch (e) {
avoidPrint(
'=========> Warning: Plugin Push Notifications Mobile And Web App Not Installed. Download here: https://wordpress.org/plugins/push-notification-mobile-and-web-app');
}
}
/// Remove user token database
Future<void> removeTokenInDatabase(
RequestHelper requestHelper,
String? token,
String? userId, {
List<String>? topics,
}) async {
try {
if (topics != null) {
await unSubscribeTopic(topic: topics);
}
} catch (e) {
avoidPrint(
'=========> Warning: Plugin Push Notifications Mobile And Web App Not Installed. Download here: https://wordpress.org/plugins/push-notification-mobile-and-web-app');
}
}
/// Get token
Future<String?> getToken() async {
return null;
}
/// Subscribing to topics
Future<void> subscribeTopic({dynamic topic, String? userId, String? email}) async {
if (kIsWeb) return;
if (topic is String) {
OneSignal.shared.sendTag("topic", topic);
}
if (topic is List<String>) {
Map<String, String> dataRoles = {};
for (var item in topic) {
dataRoles.putIfAbsent(item, () => "1");
}
OneSignal.shared.sendTags({"user_id": userId, "email": email, ...dataRoles});
}
}
/// Un subscribing to topics
Future<void> unSubscribeTopic({dynamic topic}) async {
if (kIsWeb) return;
if (topic is List<String>) {
OneSignal.shared.deleteTags(["user_id", "email", ...topic]);
avoidPrint("Un subscribing to topics $topic");
}
}
/// Listening the changes
mixin MessagingMixin<T extends StatefulWidget> on State<T> {
Future<void> subscribe(RequestHelper requestHelper, Function navigate) async {
OneSignal.shared.setNotificationOpenedHandler((openedResult) {
Map<String, dynamic>? additionalData = openedResult.notification.additionalData;
Map<String, dynamic> data = {
'type': additionalData!['type'],
'route': additionalData['route'],
'args': jsonDecode(additionalData['args'])
};
navigate(data);
});
}
}
Note: After the setup is complete, delete the application on the device before running it again.